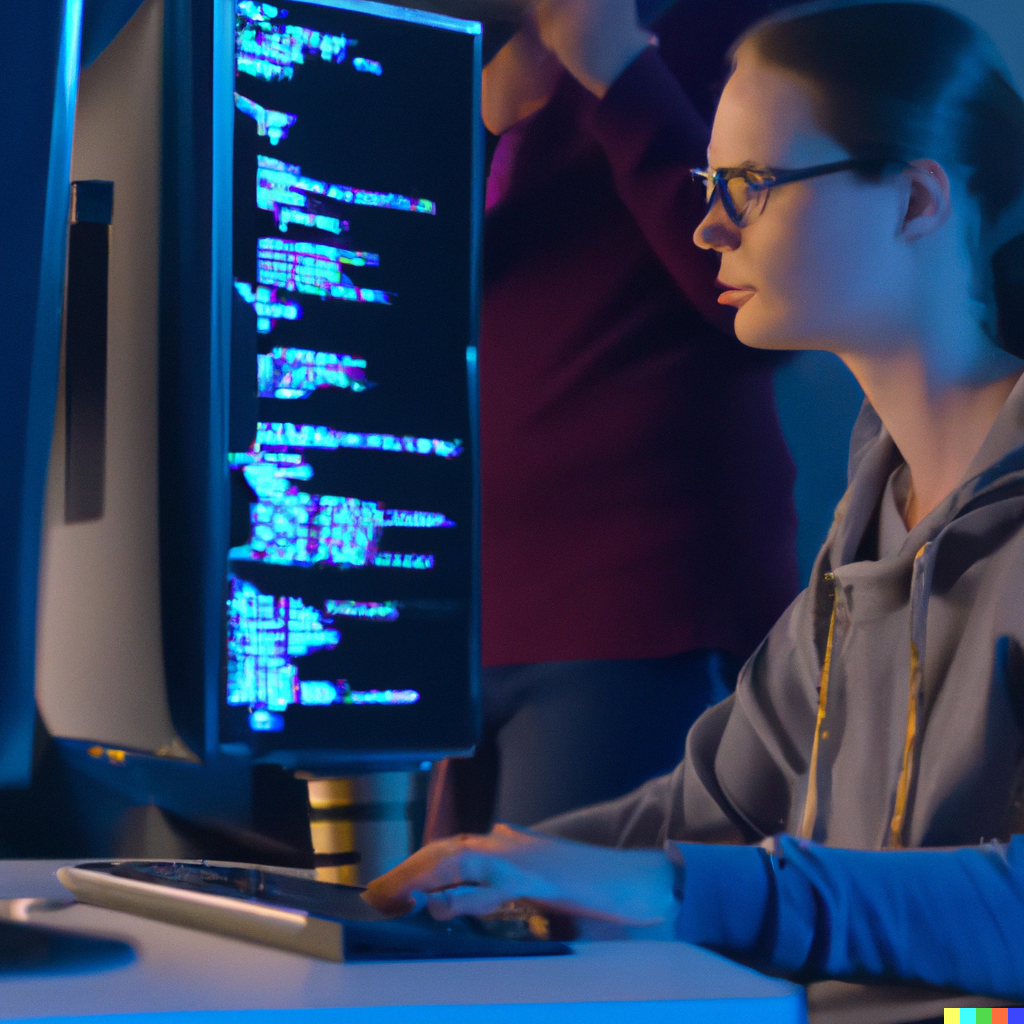
Algorithms are the backbone of software engineering and every software engineer should have a good understanding of the most common and important algorithms. Here is a list of top 10 algorithms that every software engineer should know by heart:
- Sorting algorithms (e.g. quicksort, mergesort, heapsort)
- Search algorithms (e.g. binary search, breadth-first search, depth-first search)
- Hash table algorithms (e.g. open addressing, chaining, consistent hashing)
- Graph algorithms (e.g. Dijkstra’s shortest path, Bellman-Ford, A*)
- Dynamic programming algorithms (e.g. longest common subsequence, knapsack problem, matrix chain multiplication)
- Greedy algorithms (e.g. Huffman coding, Prim’s minimum spanning tree, Dijkstra’s shortest path)
- Divide and conquer algorithms (e.g. merge sort, quicksort, Karatsuba algorithm)
- Backtracking algorithms (e.g. n-queens problem, Sudoku solver, m-coloring problem)
- Randomized algorithms (e.g. randomized quicksort, randomized median finding, randomized algorithms for graph properties)
- Compression algorithms (e.g. Huffman coding, LZ77, LZ78, LZW)
Please note, this list can also be influenced by the field of software engineering you are working in and which topics are more related to that specific field. However, this list can give you a good general overview of some of the algorithms that software engineers should know by heart.